Renta Javascript SDK
Renta JavaScript SDK enables you to collect data from a website and stream customer behavioral data to various destinations:
Snowflake
Google BigQuery
Clickhouse
Amazon Redshift
Amazon S3
Facebook Conversions API
Webhooks
This data source's advantage is its ability to support server-side integration, which collects data on behalf of the first-party data.
Below is a detailed guide to help you set up data tracking on your website.
Setup Renta Javascript SDK
Select Renta Javascript SDK in the integration Catalog to create a new data source.
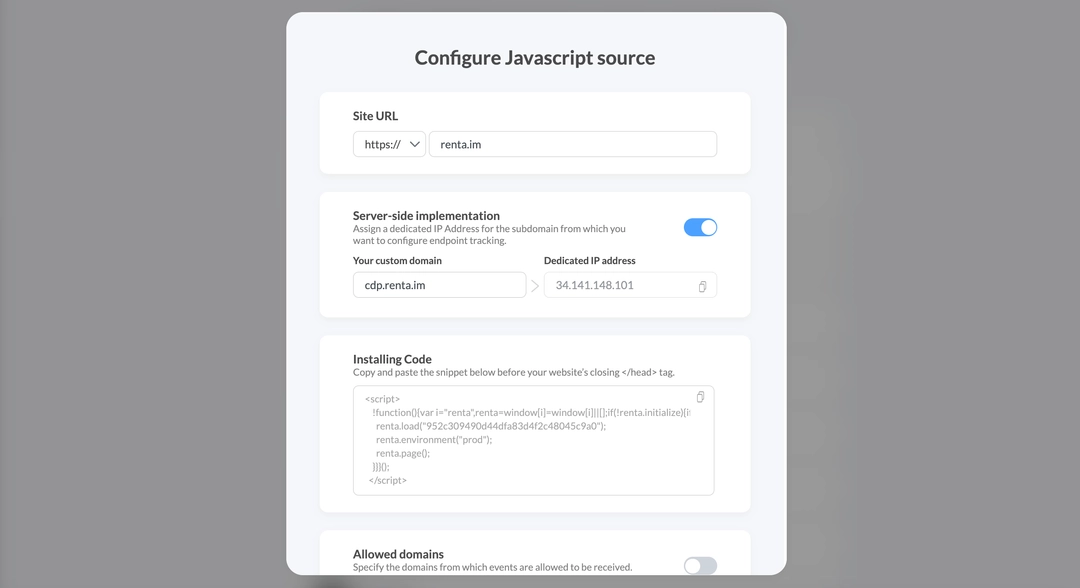
Then specify the following settings:
Site URL
Specify the URL of the site for which you want to set up tracking.
Server-side implementation
Allows you to configure server-side tracking. By default, tracking is done by Renta, but we strongly recommend using a subdomain of your website.
In order to do this, enable server-side implementation and specify the following settings:
Your custom domain
Enter the subdomain of your website on behalf of which the tracking will take place in the Custom domain field. For example: collect.yourdomain.com.Dedicated IP address
Copy the dedicated IP address of your project's server and set it as the A-record for the specified subdomain. If you do not perform the configuration by yourself, then use the instructions for the IT department.
Installing code
Copy the received code into the Installing Code field and install it on all site pages, for instance, using Google Tag Manager.
However, we strongly recommend adding the code directly to your site because Google Tag Manager is often blocked by various browsers, which can prevent data from being tracked accurately.
Environment settings
The Renta JavaScript SDK allows you to specify the environment from which events will be sent, such as production or development.
This feature is particularly useful for differentiating between different environments and managing data more efficiently.
Specifying an environment helps you to:
Separate data: easily distinguish data from different environments, such as production and development.
Improve Data Quality: ensure that test or development data is not mixed with production data.
Simplify Debugging: quickly identify environment-specific problems.
Filter and block events: filter or block events based on the specified environment, providing more precise control over data collection.
Add an environment
To specify an environment, add the setting renta.environment() to your Renta tracking snippet. Here's how to modify the Renta tracking snippet to include the environment parameter with the value «prod»:
<script>
!function(){var i="renta",renta=window[i]=window[i]||[];if(!renta.initialize){if(renta.invoked){window.console&&console.error&&console.error("Renta SDK snippet included twice.");}else{renta.invoked=true;renta.methods=["identify","group","track","page"];renta.factory=function(method){return function(){if(window[i].initialized)return window[i][method].apply(window[i],arguments);var args=Array.prototype.slice.call(arguments);args.unshift(method);renta.push(args);return renta;};};for(var n=0;n<renta.methods.length;n++){var key=renta.methods[n];renta[key]=renta.factory(key);}renta.environment=function(env){renta.env=env;};renta.load=function(key,domain){renta.writeKey=key;renta.collectURL=domain||'cdp.renta.im';var script=document.createElement("script");script.type="text/javascript";script.async=true;script.src="https://"+renta.collectURL+"/collect/v2/renta.min.js";var first=document.getElementsByTagName("script")[0];first.parentNode.insertBefore(script,first);};renta.SNIPPET_VERSION="2.1";
renta.load("YOUR_TRACKING_CODE");
renta.environment("prod");
}}}();
</script>
Renta provides snippet code with this parameter by default, but it is optional. You can remove it, in which case all events will be sent with the environment set to null.
Now you can go to the event settings on the site side.
Configuring Event Tracking
Using the Javascript SDK, you can configure 4 types of events:
Event type | Description |
---|---|
page | Captures information about page views. |
track | Provides tasks for tracking user actions. For instance to track transactions or subscribe to a newsletter. |
identity | Recommended to use only to collect users’ personal data. Helps to build a user profile. |
group | The event is used to track a group of users. |
Page
This is the basic event that sends information about the page view. Recommended to call this event on all pages of the site.
To call a page view event, use the code below:
<script>
renta.page()
</script>
You can also specify additional attributes if the standard data is not enough.
Track
Use it to record any user actions on the site. You can create custom event names with any number of descriptive attributes.
You can see the scenarios for using this type of event below:
Registration in the product;
Subscribe to the newsletter;
Making a transaction in the online store;
Thus, you can create any events that are valuable for your project.
Example of calling javascript code to commit a transaction:
<script>
renta.track('Transaction completed', {
transactionId: "ABC00001",
transactionRevenue: 1000
});
</script>
Each track event consists of:
Field name | Mandatory | Field description |
---|---|---|
Event name | ✅ | The name of the event. For instance, Transaction completed. |
Properties | ❌ | This is any additional information that you can send along with the event. You can specify any number of additional attributes. As an example, the transactionId and transactionRevenue variables are transfered to the Transaction completed event. They describe the amount and number of order. These variables are additional attributes of the event. You can delete them, rename them, or specify your own attributes. |
Identity
It is necessary to use Identity only at the moment of user identification. Below are the scenarios when one should call this event type:
After successful registration.
After successful authorization.
When the user updates their contact information.
To call the event, use the javascript code:
<script>
renta.identify("userid_variable", {
name: "Elon Musk",
email: "elon@tesla.com",
company: "Tesla Motors"
});
</script>
In addition to the standard fields, identity supports the following fields:
Fields name | Mandatory | Field description |
---|---|---|
userId | ✅ | Unique user ID in your database. |
Properties | ❌ | This is any additional information that you can send along with the event. You can specify any number of additional attributes. As an example, the javascript code contains the variables name, email, company. These variables are additional attributes of the event. You can delete them, rename them, or specify your own attributes. |
Group
The event is used to unite users into a common group.
The name of the company, department, or project can be used as a group. Thus, you can use any internal identifier in your system.
To call the event, use the javascript code:
<script>
renta.group("groupid_variable", {
company: "Tesla",
industry: "Auto Manufacturing",
employees: 70757
});
</script>
In addition to the standard fields, the group supports the following fields:
Field name | Mandatory | Field description |
---|---|---|
groupId | ✅ | Unique ID of the group in your database. |
Properties | ❌ | This is any additional information that you can send along with the event. You can specify any number of additional attributes. As an example, the javascript code contains variables company, industry, employees. These variables are additional attributes of the event. You can delete them, rename them, or specify your own attributes. |
Event version control
To add versioning to events, include the event_version parameter in the event context.
This feature enables you to better track and manage event changes over time to maintain consistency between implementations, quickly identify and fix issues, and ensure that the correct version of the event is being used.
Here is an example of an event with a version:
<script>
renta.track('Order Completed', {
subtotal: 23,
products: [{
product_name: 'Air Balloon',
product_id: '32rd9jfs'
}],
order_id: '2df90eiwc9wjec',
revenue: 33
}, {
context: {
protocols: {
event_version: '1.1'
}
}
});
</script>
In the example, the event_version value is 1.1, which indicates the version of the event currently in use.
Structure of standard fields
Initially, events of all types come with a standard list of fields that are available for transmission in server-side integrations and are also stored in a separate table during DWH integration.
The list of standard fields, as well as their description, is available in the table below:
Field name | Type of data | Description |
---|---|---|
dateTime | datetime | Date and time of the event in UTC |
date | date | Date of the event in UTC |
sendAt | datetime | Date and time when the event was sent from the client side |
eventId | String | Unique Event ID |
eventType | String | Event type |
eventName | String | Event name |
properties | String | Json object that contains custom event attributes |
anonymousId | String | Unique cookie values |
userId | String | UserId number. Contains the value passed to Google Analytics. |
groupId | String | Unique group value (only available for a group type event) |
content | String | Maintance of utm_content |
medium | String | Maintance ofutm_medium |
source | String | Maintance of utm_source |
term | String | Maintance of utm_term |
campaign | String | Maintance of utm_campaign |
dataSource | String | The data source for the request. Initialy, requests from analytics.js they will have the web value, and requests from the mobile SDK will have the app value. |
device | String | Device type: desktop, tablet, mobile, TV. |
deviceBrowser | String | Browser (for instance, Chrome or Firefox). |
deviceBrowserVersion | String | Browser version. |
deviceOperatingSystem | String | Operating system(for instance, Macintosh or Windows). |
deviceOperatingSystemVersion | String | Operating system version. |
deviceIp | String | IP-adress |
country | String | Country |
city | String | City |
region | String | Region |
deviceUserAgent | String | User Agent |
deviceScreenHeight | Int | Resolution of the user’s screen in pixels by height |
deviceScreenWidth | Int | Resolution of the user’s screen in pixels by width |
deviceViewPortHeight | Int | The size of the viewing area in the browser by height |
deviceViewPortWidth | Int | The size of the viewing area in the browser by width |
deviceDocEncoding | String | Encoding. For instance, UTF-8. |
location | String | URL with additional parameters. For instance: https://site.com/catalog/platya?page=2 |
hostname | String | Domain. For instance: https://site.com/ |
path | String | Path of the pages URL. For instance: /catalog/platya |
title | String | Title of the page. |
page | String | The full URL path. For instance: https://site.com/catalog/platya |
documentReferrer | String | If the medium field has the referral value, the path of the traffic source is indicated here, and the host name is contained in the source field. |
fbclid | String | The Facebook Ads click ID that is passed to the URL when the ad is clicked. |
gclid | String | The Google Ads click ID that is passed to the URL when the ad is clicked. |
ydclid | String | The Yandex Direct click ID that is passed to the URL when the ad is clicked. |
ga_cookie_id | String | The unique meaning of Google Analytics cookies |
ym_cookie_id | String | The unique meaning of Yandex Metrika cookies |
fbc_cookie_id | String | The unique meaning of Facebook cookies |
fbp_cookie_id | String | The unique meaning of Facebook cookies |
offset | String | A service parameter of the Javascript SDK. Used to count sessions (the session table is only available in integration with DWH). |